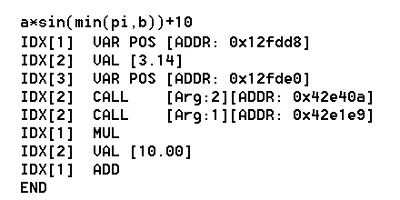
A fast math expression parser capable of parallel expression evaluation.
The evaluation of a mathematical expression is a standard task in many applications. It can be solved by using a standard math expression parser such as muparser or by embedding a scripting language such as Lua. There are however some limitations: Although muparser is pretty fast it will only work with scalar values and although Lua is very flexible it does neither support binary operators for arrays nor complex numbers. So if you need a math expression parser with support for arrays, matrices and strings muparserx may be able to help you. It was originally based on the original muparser engine but has since evolved into a standalone project with a completely new parsing engine.
Parser | Data types | Precision | User defined operators | User defined functions | Localization | Licence | Performance (Expr. per second) | ||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
complex | scalar | string | vector | Binary | Postfix | Infix | Strings as parameters | Arbitrary number of parameters | |||||
muparser | ![]() |
![]() |
![]() |
![]() |
double | ![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
BSD 2-Clause | ~ 10.000.000 |
muparserSSE | ![]() |
![]() |
![]() |
![]() |
float | ![]() |
![]() |
![]() |
![]() |
max. 10 | ![]() |
BSD 2-Clause | ~ 20.000.000 |
muparserX | ![]() |
![]() |
![]() |
![]() |
double | ![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
BSD 2-Clause | ~ 1.600.000 |
Table 1: Feature comparison with other derivatives of muparser. (* Average performance calculated using this set of expressions; (1) muparser can define strings but only as constants.)
e = 2.718281828459045235360287
pi = 3.141592653589793238462643
i = sqrt(-1)
+, -, *, /, ^
=, +=, -=, *=, /=
&&, ||, ==, !=, >, <, <=, >=
&, |, <<, >>
//
?:
n, mu, m, k, G, M
'
(for vectors and matrices)
-, (float), (int)
a[1,2]
(a<b) ? c:d
{1,2,3}
abs, sin, cos, tan, sinh, cosh, tanh, ln, log, log10, exp, sqrt
min, max, sum
str2dbl, strlen, toupper
real, imag, conj, arg, norm
sizeof, eye, ones, zeros
The next table shows samples of expressions that can be evaluated using muparserx:
Expression | Result | Explanation |
---|---|---|
"hello"=="world" | false | Comparing strings |
"hello "//"world" | "hello world" | String concatanation operator |
sin(a+8i) | ... | Support for a variety of predefined functions working with complex numbers. |
va[3]+vb[5] | ... | Support for array variables |
va[3]=9 | ... | Assignment operator in combination with indexed access to a vector |
toupper("hello"//"world") | "HELLOWORLD" | Transforming a concatenated string to uppercase. |
#010010 | 18 | Interpreting binary values |
0x1eff | 7935 | Interpreting hex values |
#10>0x1eff | false | Comparing binary and hex values |
1+2-3*4/5^6 | 2.99923 | The standard operators |
a = ((a<b) ? 10 : -10) | 10 or -10 depending on a and b | Ternary operator for if-then-else conditionals |