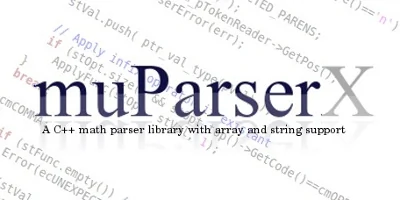
A math expression parser with support for strings, vectors and matrices.
The muparser library is using a CMake based build system. You can load the folder into an IDE that supports CMake such as Visual Studio or build the library from the command line.
cd [path to muParser]
cmake . [-DENABLE_SAMPLES=ON/OFF] [-DENABLE_OPENMP=ON/OFF] [-DENABLE_WIDE_CHAR=OFF/ON] [-DBUILD_SHARED_LIBS=ON/OFF]
make
sudo make install
sudo ldconfig
cd samples/example1
./example1
If you do not want the build files to be placed in the muparser folder i recommend creating a separate build folder:
cd [path to muParser]
mkdir build
cd build
cmake .. [-DENABLE_SAMPLES=ON/OFF] [-DENABLE_OPENMP=ON/OFF] [-DENABLE_WIDE_CHAR=OFF/ON] [-DBUILD_SHARED_LIBS=ON/OFF]
make
./example1
When this option is set the example applications will be built. There are two samples, one for demonstrating the class API (example1.cpp), the other for demonstrating the C-API example2.c.
Default is "On". When this option is set muparser is built with OpenMP support for evaluations in bulk mode. When this option is set computations in Bulk mode will be performed in parallel on all available CPU cores. This will speed up expression evaluation significantly.
Default is "Off". When this option is set, muparser will use wide character strings instead of ASCII strings. You cannot link a muparser library built with wide character support against an application using ASCII strings!
Default is "On". When this option is set mupaser will be built as a shared library.
I usually recommand embedding muparser directly into projects. Embedding the library source code directly into a client application is the easiest way to avoid linker conflicts originating from different versions of the runtime libraries used by the shared parser library and your project. In order to embed muparser include the following files into your project:
muParser.cpp
muParserBase.cpp
muParserBytecode.cpp
muParserCallback.cpp
muParserError.cpp
muParserTokenReader.cpp
And make sure the following include files can be found in your projects include path:
muParser.h
muParserBase.h
muParserBytecode.h
muParserCallback.h
muParserDef.h
muParserError.h
muParserFixes.h
muParserTemplateMagic
muParserToken.h
muParserTokenReader.h
The parser class and all related classes reside in the namespace mu, make sure to either add a using
using namespace mu;
to your files or reference all classes with their complete name.
If you use muParser by compiling your own version or including the source code directly you can use a set of preprocessor definitions in order to customize its behaviour. The following definitions are located in the file muParserDef.h:
The macro MUP_BASETYPE defines the underlying datatype used by muParser. This can be any floating point value type (float, double or long double). The macro defaults to double. Modify this value if you need higher precision or want to use muParser seemless with client code that is using float as its data type.
#define MUP_BASETYPE double
If you are using the CMake build system OpenMP support is activated with the -DENABLE_OPENMP=OFF/ON option. The option will set the macro MUP_USE_OPENMP for you and link with OpenMP automatically. When OpenMP support is enabled, expression evaluation in bulk mode will be executed in parallel on multiple CPU's. By default OpenMP support is active when building with CMake.
#define MUP_USE_OPENMP
If you are not building with CMake and have enbedded the source code directly in your project, uncomment the macro in the file muParserDef.h.
Depending on your hardware, parallelization can speed up the parser significantly (30% - 400%). Enabling parallelization does not have a significant effect on short expressions.
This definition determines the string type used by muParser. This can either be std::string or std::wstring. This definition shouldn't be set directly. It is defined to std::wstring if there is a preprocessor macro _UNICODE present.
#define MUP_STRING_TYPE std::wstring