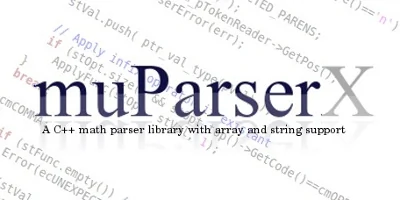
A math expression parser with support for strings, vectors and matrices.
sin(x), x+y, x*x
abc=123
You can define how a value looks like. You can make muparser to read hex values like a=0x00ff00
or
binary values. a=0b1000101
. You could also use this feature to Query values from a database.
The following table gives an overview of the functions supported by the default implementation. It lists the function names, the number of arguments and a brief description.
Name | Argc. | Explanation |
rnd | 0 | Generate a random number between 0 and 1 |
sin | 1 | sine function |
cos | 1 | cosine function |
tan | 1 | tangens function |
asin | 1 | arcus sine function |
acos | 1 | arcus cosine function |
atan | 1 | arcus tangens function |
sinh | 1 | hyperbolic sine function |
cosh | 1 | hyperbolic cosine |
tanh | 1 | hyperbolic tangens function |
asinh | 1 | hyperbolic arcus sine function |
acosh | 1 | hyperbolic arcus tangens function |
atanh | 1 | hyperbolic arcur tangens function |
log2 | 1 | logarithm to the base 2 |
log10 | 1 | logarithm to the base 10 |
log | 1 | logarithm to base e (2.71828...) |
ln | 1 | logarithm to base e (2.71828...) |
exp | 1 | e raised to the power of x |
sqrt | 1 | square root of a value |
sign | 1 | sign function -1 if x<0; 1 if x>0 |
rint | 1 | round to nearest integer |
abs | 1 | absolute value |
min | var. | min of all arguments |
max | var. | max of all arguments |
sum | var. | sum of all arguments |
avg | var. | mean value of all arguments |
The following table lists the default binary operators supported by the parser.
Operator | Description | Priority |
= | assignement * | 0 |
|| | logical or | 1 |
&& | logical and | 2 |
| | bitwise or | 3 |
& | bitwise and | 4 |
<= | less or equal | 5 |
>= | greater or equal | 5 |
!= | not equal | 5 |
== | equal | 5 |
> | greater than | 5 |
< | less than | 5 |
+ | addition | 6 |
- | subtraction | 6 |
* | multiplication | 7 |
/ | division | 7 |
^ | raise x to the power of y | 8 |
muparser has built in support for the if then else operator. It uses lazy evaluation in order to make sure only the necessary branch of the expression is evaluated.
Operator | Description | Remarks |
?: | if then else operator | C++ style syntax |
The names of predefined constant names are prefixed with an underscore. The parser has two predefined constants: Pi and Eulers number. The accuracy of the constant definition ultimately depends on the size of the selected value type (float, double, long double).
Constant | Description | Remarks |
_pi | The one and only pi. | 3.141592653589793238462643 |
_e | Euler's number. | 2.718281828459045235360287 |