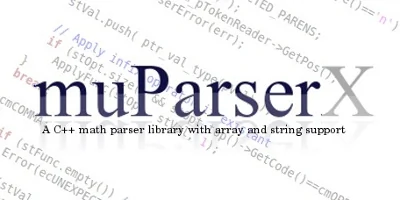
A math expression parser with support for strings, vectors and matrices.
In case of an error both parser class and the parser DLL provide similar methods for querying the information associated with the error. In the parser class they are member functions of the exception class mu::Parser::exception_type and in the DLL version they are normal functions. These functions are:
The following table lists the parser error codes. The first column contains the enumeration values as defined in the enumeration mu::EErrorCodes located in the file muParserError.h. Since they are only accessible from C++ the second column lists their numeric code and the third column contains the error description.
Enumeration name | Value | Description |
ecUNEXPECTED_OPERATOR | 0 | Unexpected binary operator found |
ecUNASSIGNABLE_TOKEN | 1 | Token cant be identified |
ecUNEXPECTED_EOF | 2 | Unexpected end of formula. (Example: "2+sin(") |
ecUNEXPECTED_ARG_SEP | 3 | An unexpected argument separator has been found. (Example: "1,23") |
ecUNEXPECTED_ARG | 4 | An unexpected argument has been found |
ecUNEXPECTED_VAL | 5 | An unexpected value token has been found |
ecUNEXPECTED_VAR | 6 | An unexpected variable token has been found |
ecUNEXPECTED_PARENS | 7 | Unexpected parenthesis, opening or closing |
ecUNEXPECTED_STR | 8 | A string has been found at an inapropriate position |
ecSTRING_EXPECTED | 9 | A string function has been called with a different type of argument |
ecVAL_EXPECTED | 10 | A numerical function has been called with a non value type of argument |
ecMISSING_PARENS | 11 | Missing parens. (Example: "3*sin(3") |
ecUNEXPECTED_FUN | 12 | Unexpected function found. (Example: "sin(8)cos(9)") |
ecUNTERMINATED_STRING | 13 | unterminated string constant. (Example: "3*valueof("hello)") |
ecTOO_MANY_PARAMS | 14 | Too many function parameters |
ecTOO_FEW_PARAMS | 15 | Too few function parameters. (Example: "ite(1<2,2)") |
ecOPRT_TYPE_CONFLICT | 16 | binary operators may only be applied to value items of the same type |
ecSTR_RESULT | 17 | result is a string |
ecINVALID_NAME | 18 | Invalid function, variable or constant name. |
ecINVALID_BINOP_IDENT | 19 | Invalid binary operator identifier. |
ecINVALID_INFIX_IDENT | 20 | Invalid infix operator identifier. |
ecINVALID_POSTFIX_IDENT | 21 | Invalid postfix operator identifier. |
ecBUILTIN_OVERLOAD | 22 | Trying to overload builtin operator |
ecINVALID_FUN_PTR | 23 | Invalid callback function pointer |
ecINVALID_VAR_PTR | 24 | Invalid variable pointer |
ecEMPTY_EXPRESSION | 25 | The expression string is empty |
ecNAME_CONFLICT | 26 | Name conflict |
ecOPT_PRI | 27 | Invalid operator priority |
ecDOMAIN_ERROR | 28 | catch division by zero, sqrt(-1), log(0) (currently unused) |
ecDIV_BY_ZERO | 29 | Division by zero (currently unused) |
ecGENERIC | 30 | Error that does not fit any other code but is not an internal error |
ecLOCALE | 31 | Conflict with current locale |
ecUNEXPECTED_CONDITIONAL | 32 | Unexpected if then else operator |
ecMISSING_ELSE_CLAUSE | 33 | Missing else clause |
ecMISPLACED_COLON | 34 | Misplaced colon |
ecUNREASONABLE_NUMBER_OF_COMPUTATIONS | 35 | The vectors submitted to bulk mode computations are too short. |
ecIDENTIFIER_TOO_LONG | 36 | A submitted identifier longer than 100 characters. |
ecEXPRESSION_TOO_LONG | 37 | The submitted expression is longer than 5000 characters. |
ecINVALID_CHARACTERS_FOUND | 38 | Internal error of any kind. |
ecINTERNAL_ERROR | 39 | Internal error of any kind. |
Since dynamic libraries with functions exported in C-style can't throw exceptions the DLL version provides the user with a callback mechanism to raise errors. Simply add a callback function that does the handling of errors. Additionally you can query the error flag with mupError(). Please note that by calling this function you will automatically reset the error flag!
// Callback function for errors
void OnError(muParserHandle_t hParser)
{
printf("\nError:\n");
printf("------\n");
printf("Message: \"%s\"\n", mupGetErrorMsg(hParser));
printf("Token: \"%s\"\n", mupGetErrorToken(hParser));
printf("Position: %d\n", mupGetErrorPos(hParser));
printf("Errc: %d\n", mupGetErrorCode(hParser));
}
...
// Set a callback for error handling
mupSetErrorHandler(hParser, OnError);
// The next function could raise an error
fVal = mupEval(hParser);
// Test for the error flag
if (!mupError())
{
printf("%f\n", fVal);
}
See also: example2/example2.c
In case of an error the parser class raises an exception of type Parser::exception_type
. This
class provides you with several member functions that allow querying the exact cause as well as
additional information for the error.
try
{
...
parser.Eval();
...
}
catch(mu::Parser::exception_type &e)
{
cout << "Message: " << e.GetMsg() << "\n";
cout << "Formula: " << e.GetExpr() << "\n";
cout << "Token: " << e.GetToken() << "\n";
cout << "Position: " << e.GetPos() << "\n";
cout << "Errc: " << e.GetCode() << "\n";
}
See also: example1/example1.cpp